Mastering Git: Essential Command-Line for Developers
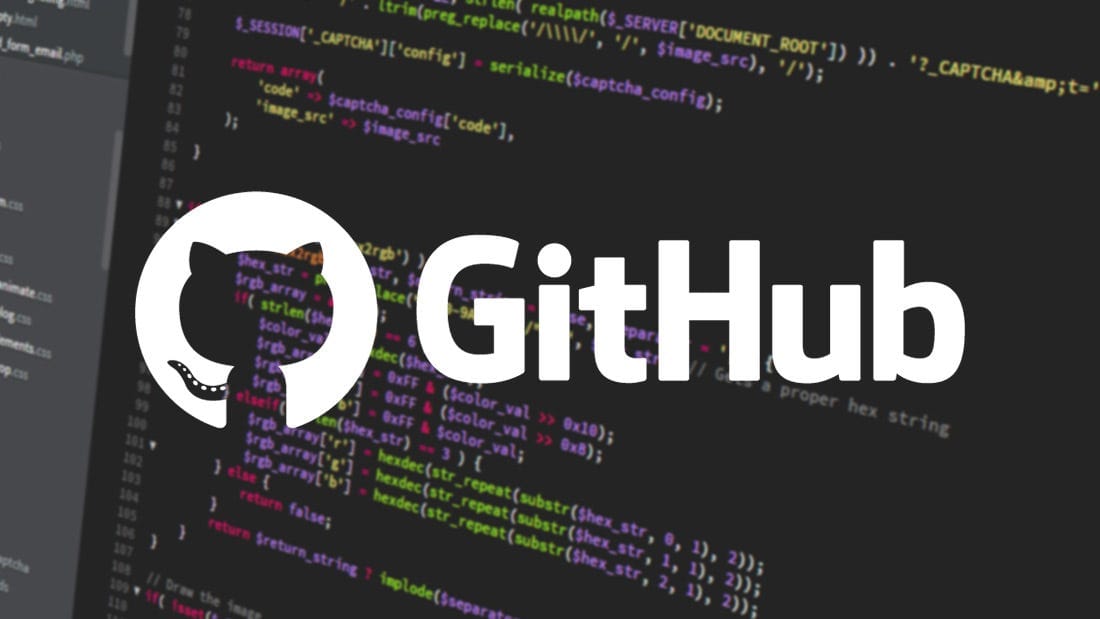
Git is an indispensable version control tool that streamlines collaboration and code management for developers. While graphical user interface (GUI) tools can simplify some tasks, mastering the Git command line unlocks deeper control, flexibility, and speed. Here are 20 Git command-line tricks that every developer should know to enhance their workflow.
1. Set Global Configuration
Ensure your commits reflect your correct identity by setting up your global configuration:
git config --global user.name "Your Name"
git config --global user.email "[email protected]"
--local
instead of --global
to configure settings for a specific project.2. Undo the Last Commit Without Losing Changes ( Not Pushed )
If you made a mistake in your last commit, you can revert it without losing your changes:
git reset --soft HEAD~1
HEAD~1 means "the parent of HEAD
," which is the commit immediately before the current commit.
3. Undo the Last Commit Without Losing Changes (Pushed Commit)
git reset
as it rewrites history and may disrupt others working on the branch. Use git revert
to creates a new commit that undoes the previous changes while keeping the commit history intact.
Steps to Undo a Pushed Commit Without Losing Changes:
- Identify the hash of the commit you want to undo (the last commit in this case):
git log
- Revert the commit:
git revert <commit-hash>
This will open a text editor for the commit message. You can modify the message or leave it as is.
- Push the revert commit to the remote repository:
git push
Key Benefits of git revert
- Keeps the commit history intact.
- Avoids the need for a force push (
git push --force
). - Safely undoes changes without impacting others working on the branch.
Example
If your last commit hash is abc123
, run:git revert abc123
git push
This approach is the safest for undoing changes in a collaborative environment.
4. Amend the Last Commit (Not Pushed)
Did you forget to include a change or need to update your commit message? You can easily amend the last commit with the following commands:
git add .
git commit --amend -m "Updated commit message"
Allows you to replace the commit message with a new one
This command updates the previous commit without creating a new one.
5. Stash Uncommitted Changes
If you need to quickly switch branches without committing your changes, use:
git stash
You can retrieve your stashed changes later with:
git stash pop
6. View Commit History Graphically
Visualizing your commit history can make it easier to understand the project's state:
git log --graph --oneline --all
7. Change the Commit Author (Not Pushed)
Need to update the author of the last commit? It's easy to do:
git commit --amend --author="New Author <[email protected]>"
8. Check Differences in Staged Changes
Use git diff
to compare staged changes that haven’t yet been committed:
git diff --staged
9. Find a Bug with Bisect
Use git bisect
to find the commit that introduced a bug:
git bisect start
git bisect bad # Mark the current commit as bad
git bisect good <commit-hash> # Mark a known good commit
This command will guide you through the history to identify the problematic commit.
10. Rebase for a Clean Commit History
Rebasing can help you rewrite commit history for better clarity:
git rebase -i HEAD~3
This lets you edit, squash, or reorder your last 3 commits.
11. Cherry-Pick Specific Commits
Want to incorporate a specific commit from another branch? Use:
git cherry-pick <commit-hash>
12. List All Branches (Local and Remote)
To see all available branches, local and remote, run:
git branch -a
13. Clean Untracked Files and Directories
Quickly remove unwanted files that are not tracked by Git with:
git clean -fd
đź’ˇ Tip: Use -n
for a dry run to preview the files that will be removed.
14. Track an Upstream Branch
To keep your local branch in sync with a remote branch, set it upstream:
git branch --set-upstream-to=origin/main
15. Squash Commits with Interactive Rebase
Combine multiple commits into one for a cleaner history:
git rebase -i HEAD~n
Replace n
with the number of commits you wish to squash.
16. View the File at a Specific Commit
If you need to check a file's state at a particular commit:
git show <commit-hash>:path/to/file
17. Edit the .gitignore After Committing
If you forgot to ignore certain files, update your .gitignore
and remove these files from tracking:
echo "node_modules/" >> .gitignore
git rm -r --cached node_modules/
git commit -m "Update .gitignore"
18. Fetch Only Metadata
Avoid fetching the entire repository by checking what data would be fetched without downloading it:
git fetch --dry-run
19. Blame a Line of Code
Curious about who wrote a specific line in a file? Use:
git blame path/to/file
20. Reset a File to the Last Commit
To discard local changes to a specific file, run:
git checkout -- path/to/file
Conclusion
These essential Git command-line tricks are designed to enhance your development process, whether you're working solo or as part of a team. While GUI tools can offer convenience, mastering the Git command line gives you greater control over your workflows. Give these commands a try and elevate your Git skills!
Do you enjoy this blog post?